selenium 是一个 web 自动化工具,它可以控制 chrome 浏览器实现我们想要的功能,跟爬虫不同的是:它是模拟人类的操作。
安装
下载对应版本的 chromedriver
https://chromedriver.chromium.org/downloads放到 PATH 环境变量里, 如果是 mac,可以直接执行
1
| brew install chromedriver
|
安装 python 包
1
| pip install selenium -i https://pypi.tuna.tsinghua.edu.cn/simple
|
编写脚本
获取 xpath
获取 xpath 可以按下 ctrl + shift + c
点击按钮, 高亮的地方右键复制 full xpath
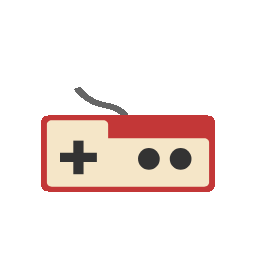
获取 id
Charome 浏览器界面按下 ctrl + shift + c
点击页面, 右边属性记录一下 html 的 id 属性
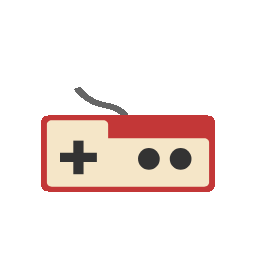
代码
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
|
import time from selenium import webdriver
driver = webdriver.Chrome() driver.get('https://gitee.com/login')
driver.maximize_window()
driver.find_element(By.ID, r'user_login').send_keys("这里写你的用户名")
driver.find_element(By.XPATH, r'/html/body/div[2]/div[1]/div[2]/div/div/div[1]/div[2]/div/div/div[2]/div/div/div/form/div[5]/button').click()
driver.quit()
|
常用操作
浏览器 options 参数
新版本 webdriver.Chrome
已经没有 chromeoptions
这个参数了。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33
| import time from selenium import webdriver from selenium.webdriver.common.by import By
options = webdriver.ChromeOptions()
options.add_experimental_option("detach", True)
options.add_argument('--headless') options.add_argument('--disable-gpu')
options.add_experimental_option('excludeSwitches', ['enable-automation']) options.add_argument('--disable-blink-features=AutomationControlled')
driver = webdriver.Chrome(options=options)
driver.execute_cdp_cmd("Page.addScriptToEvaluateOnNewDocument", { "source": """ Object.defineProperty(navigator, 'webdriver', { get: () => undefined }) """ })
driver.get('https://signin.aliyun.com/login.htm#/main')
|
截图
1
| driver.get_screenshot_as_file("test.png")
|
使用代理
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| from selenium import webdriver from selenium.webdriver import ChromeOptions
options = webdriver.ChromeOptions()
options.add_argument('--proxy-server=http://localhost:80')
options.add_argument("--ignore-certificate-errors")
options.add_experimental_option("detach", True)
driver = webdriver.Chrome(options=options)
|